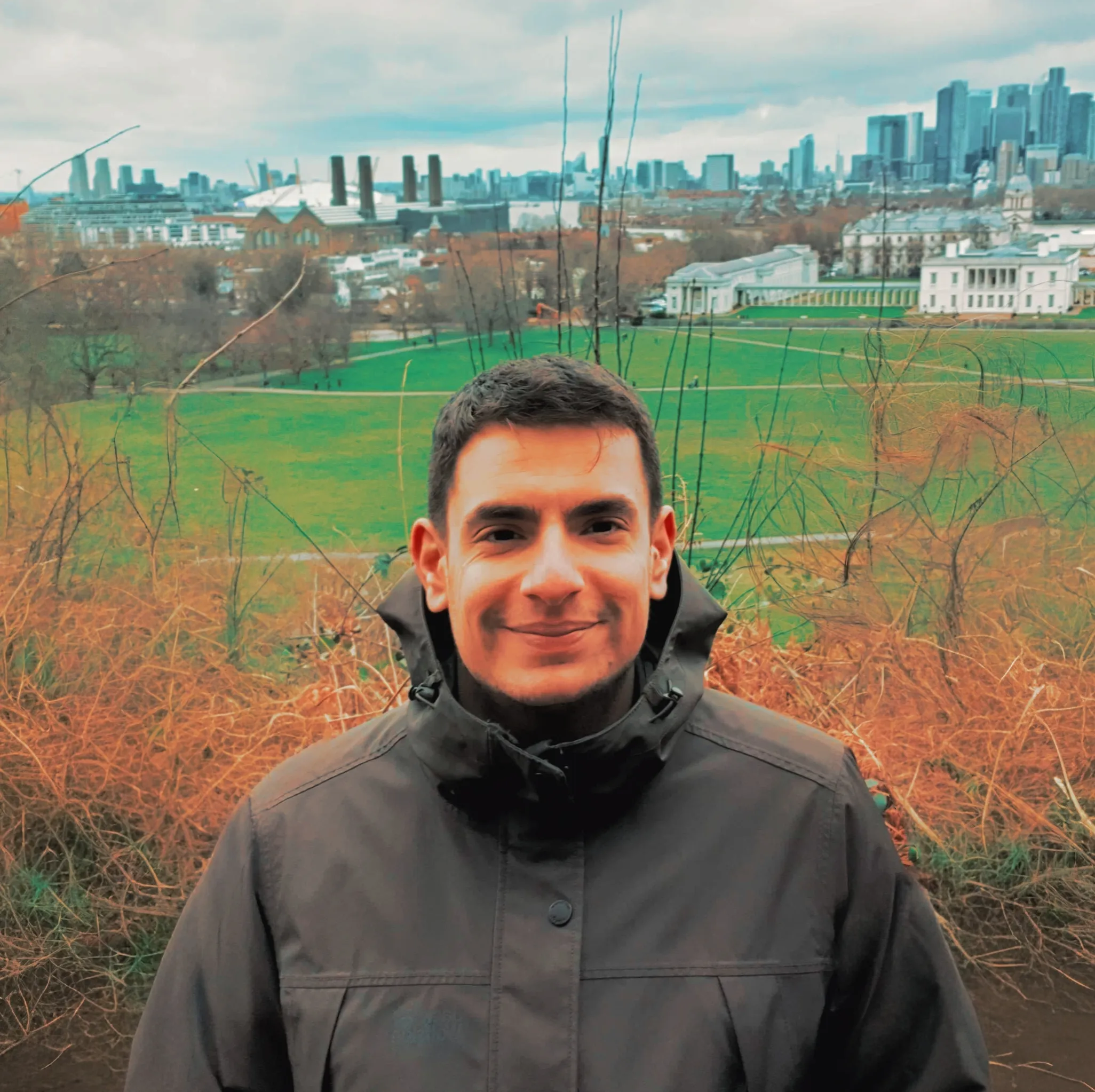
Hey 👋, I'm a software engineer based in Athens with experience in building things on the web and beyond. I am currently working at Skroutz.
This is a place where I share ideas, experiments, and things I find interesting. It also demonstrates some of my work and the stuff i am thrilled or curious about.
You can also find me here: